Summary: in this tutorial, you’ll learn how to use the JavaScript strockRect()
method to draw an outlined rectangle on a canvas.
Introduction to the JavaScript strokeRect() method
The strokeRect()
is a method of the 2D drawing context. The strokeRect()
allows you to draw an outlined rectangle with the stroke style derived from the current strokeStyle
property.
The following shows the syntax of the strokeRect()
method:
ctx.strokeRect(x, y, width, height);
Code language: CSS (css)
In this syntax:
x
is the x-axis coordinate of the starting point of the rectangle.y
is the y-axis coordinate of the starting point of the rectangle.width
is the rectangle’s width. It can be positive or negative. If thewidth
is positive, the method draws the rectangle from (x,y) to the right. Otherwise, it draws the rectangle from the (x,y) to the left.height
is the rectangle’s height. And the height can also be positive or negative. If it’s positive, the method draws the rectangle from the(x,y)
to the bottom. Otherwise, it draws the rectangle from the(x,y)
to the top.
The strokeRect()
draws directly to the canvas without modifying the current path. It means that any subsequent fill()
or stroke()
call will have no effect.
The JavaScript strokeRect() example
The following shows the index.html
page that contains a canvas element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript strokeRect</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1>JavaScript strokeRect() Demo</h1>
<canvas id="canvas" height="400" width="500">
</canvas>
<script src="js/app.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
In the app.js file, define a function that draws two outlined rectangles:
function drawOutlinedRects() {
const canvas = document.querySelector('#canvas');
if (!canvas.getContext) {
return;
}
const ctx = canvas.getContext('2d');
ctx.strokeStyle = 'red';
ctx.strokeRect(100, 100, 150, 100);
ctx.strokeStyle = 'green';
ctx.strokeRect(200, 150, -150, -100);
}
drawOutlinedRects();
Code language: JavaScript (javascript)
The following picture shows the output:
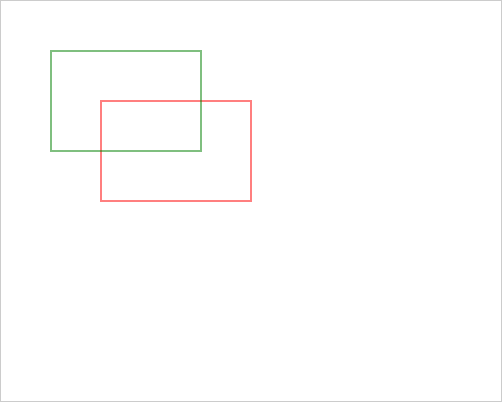
Here is the strokeRect() demo.
How it works.
- First, select the canvas element by using the
querySelector()
method. - Next, check if the browser supports the canvas API.
- Then, get the 2D drawing context from the canvas element.
- After that, set the stroke style to
red
and use thestrokeRect()
method to draw the first outline rectangle. - Finally, set the stroke style to green and draw the second outlined rectangle. In this case, we passed the negative width and height to the
strokeRect()
method to draw the rectangle to the left and top from its starting point.
Summary
- Use the
strokeRect()
method to draw an outlined rectangle starting at (x, y) with a specified width and height.