To get the closest element by a selector, you use the element.closest()
method:
const closestElement = targetElement.closest(selector);
Code language: JavaScript (javascript)
The closest()
method starts with the targetElement
and travel up to the DOM tree until it finds the element that matches the selector
. The method returns null
if no such element exists.
See the following HTML document:
<html>
<head>
<title>JavaScript closest() example</title>
</head>
<body>
<ul id="one" class="level-1">
<li class="top-1">Home</li>
<li id="ii" class="top-2">Products
<ul class="level-2">
<li class="category-1">Clothing</li>
<li class="category-2">Electronics
<ul class="level-3">
<li class="product-1">Camera</li>
<li class="product-2">Computer</li>
<li class="product-3">Phone</li>
</ul>
</li>
<li class="category-3">Kitchen</li>
</ul>
</li>
<li class="top-3">About</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
The following code selects the list item with the class category-1 and changes the color of the list item to red:
// start from the category-1
const e = document.querySelector("li.category-1");
e.style.color = 'red';
Code language: JavaScript (javascript)
The following code selects the closest <ul>
element of the selected element and changes the background of the selected element to yellow:
// highlight the closet element
const closet = e.closest("ul");
closet.style.background = 'yellow';
Code language: JavaScript (javascript)
The following picture shows the output:
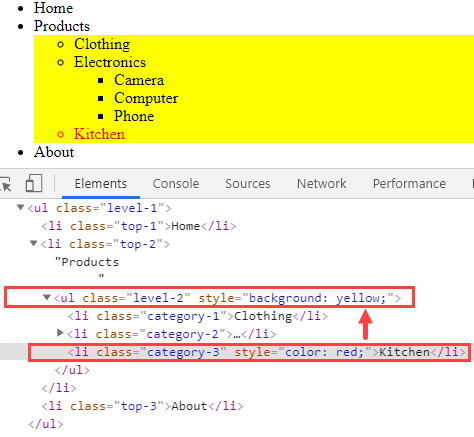
The following selects the closest list item element. The closest() method starts with the target element. Since the target element matches with the selector, the closest() method stops searching:
// start from the category-1
const e = document.querySelector("li.category-3");
e.style.color = 'red';
// highlight the closet element
const closet = e.closest("li");
closet.style.background = 'yellow';
Code language: JavaScript (javascript)
Output:
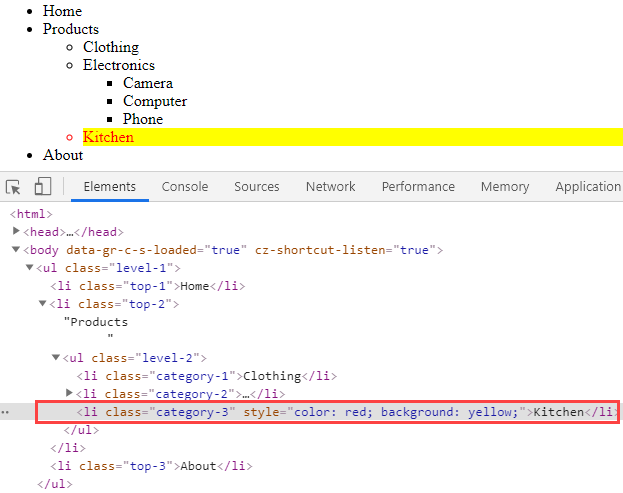