To replace a DOM element in the DOM tree, you follow these steps:
- First, select the DOM element that you want to replace.
- Then, create a new element.
- Finally, select the parent element of the target element and replace the target element by the new element using the
replaceChild()
method.
See the following HTML document:
<html>
<head>
<title>Replace a DOM element</title>
</head>
<body>
<ul>
<li><a href="/home">Home</a></li>
<li><a href="/service">Service</a></li>
<li><a href="/about">About</a></li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
To select the last list item element, you follow the above steps:
First, select the last list item using the querySelector() method:
const listItem = document.querySelector("li:last-child");
Code language: JavaScript (javascript)
Second, create a new list item element:
const newItem = document.createElement('li');
newItem.innerHTML = '<a href="/products">Products</a>';
Code language: JavaScript (javascript)
Finally, get the parent of the target element and call the replaceChild() method:
listItem.parentNode.replaceChild(newItem, listItem);
Code language: CSS (css)
Here is the output:
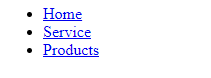
Was this tutorial helpful ?